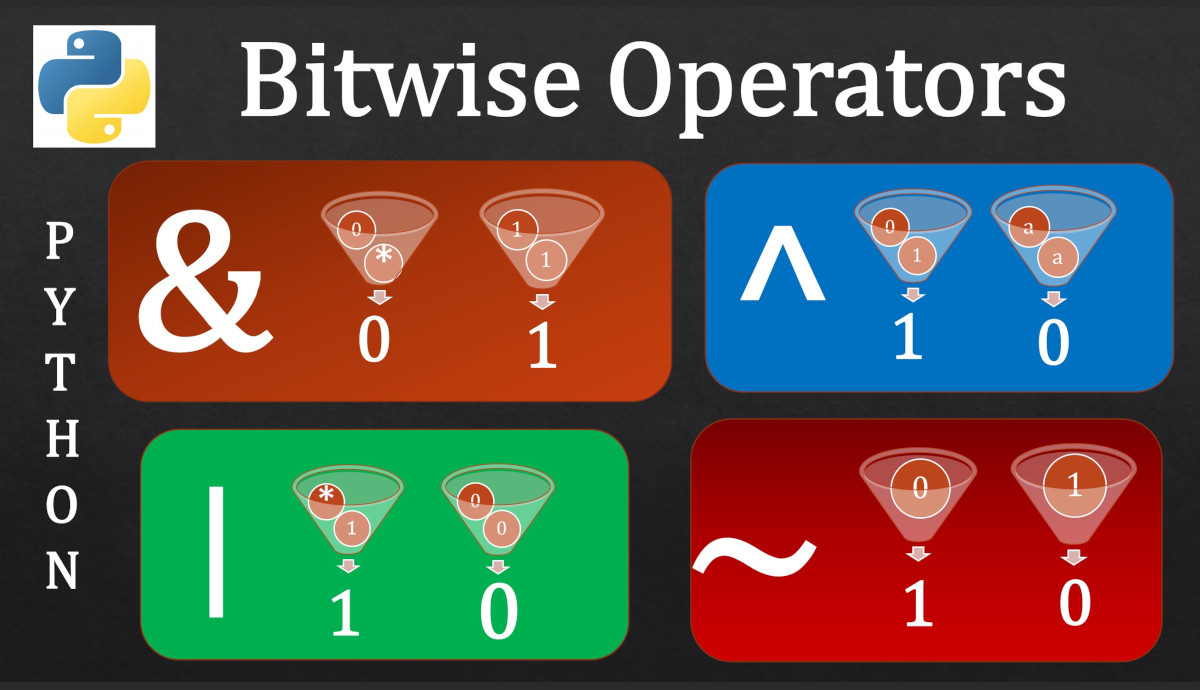
When delving into the realm of bitwise operators in Python, one is immediately introduced to a powerful set of tools that allow for fine-grained manipulation of individual bits within binary numbers. The concept itself may seem intricate at first, but as the layers are peeled back, a sense of awe and appreciation for the versatility of these operators begins to take hold. Whether it is performing logical operations, optimizing memory usage, or implementing algorithms that rely on bit-level manipulations, the world of bitwise operators in Python opens up new avenues for crafting efficient and elegant code. Brace yourself for an exploration of the intricacies and applications of these operators that will undoubtedly leave a lasting impression on your programming journey.
Table of Contents
- Introduction
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise NOT (~)
- Left Shift (<<)
- Right Shift (>>)
- Conclusion
Introduction
Bitwise operators are used to manipulate individual bits of binary numbers. In Python, there are six bitwise operators: AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
Bitwise AND (&)
The bitwise AND operator compares the corresponding bits of two numbers and returns 1 if both bits are 1, otherwise it returns 0.
Bitwise OR (|)
The bitwise OR operator compares the corresponding bits of two numbers and returns 1 if at least one of the bits is 1, otherwise it returns 0.
Bitwise XOR (^)
The bitwise XOR operator compares the corresponding bits of two numbers and returns 1 if the bits are different, otherwise it returns 0.
Bitwise NOT (~)
The bitwise NOT operator inverts all the bits of a number. It returns the one's complement of the number, meaning that each 1 becomes 0 and each 0 becomes 1.
Left Shift (<<)
The left shift operator shifts the bits of a number to the left by a specified number of positions. It effectively multiplies the number by 2 to the power of the shift amount.
Right Shift (>>)
The right shift operator shifts the bits of a number to the right by a specified number of positions. It effectively divides the number by 2 to the power of the shift amount and discards the fractional part.
Conclusion
Bitwise operators in Python provide a way to manipulate individual bits of binary numbers. They are useful in various applications such as optimizing memory usage, performing low-level operations, and implementing algorithms that require bit manipulation. Understanding how bitwise operators work and their applications can help you write more efficient and optimized code.
Operator | Description |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise XOR |
~ | Bitwise NOT |
<< | Left Shift |
>> | Right Shift |
0 Comments